The SharePoint rich text editor provides users with basic text formatting options out of the box.
However, you may want additional functionality like buttons to add custom templates, insert brand assets, or apply specialized formatting.
Fortunately, it’s straightforward to augment the rich text editor by adding custom buttons with JavaScript.
We’ll walk through the whole process step-by-step in this article. By the end, you’ll know how to configure and code custom buttons that insert content into the editor with the click of a mouse!
Prerequisites
Before adding custom buttons to the rich text editor, make sure you have:
- SharePoint Online – Will not work with SharePoint Server
- Permission to add and customize Web Parts
Some JavaScript knowledge is helpful but not required. We’ll provide code snippets you can reuse as-is.
Step 1: Add a Content Editor Web Part
The first step is to add a Content Editor Web Part (CEWP) to the page with the rich text editor. The CEWP allows executing custom JavaScript that modifies other Web Parts on that page.
Here are the basic steps to add a CEWP:
- Edit the page
- Select “Add a Web Part” from the Web Part toolbar
- Choose the Content Editor Web Part
- Set its location below the rich text editor
Step 2: Reference Editor JavaScript Library
Next, reference the rich text editor’s JavaScript library in the CEWP. This library allows programmatically interacting with the editor through JavaScript.
Here are the necessary script references:
Step 3: Define Custom Button JavaScript
Finally, we’ll write JavaScript to define the custom button and insert content when clicked:
var customButton = new CustomButton({
name: “Insert Custom Text”,
icon: “Globe”,
onClick: function() {
SP.SOD.executeOrDelayUntilScriptLoaded(insertCustomText, ‘sp.richtext.js’);
}
});
function insertCustomText() {
var editor = SP.RTE.GetActiveEditor();
editor.insertHtml(“This is custom text inserted!”);
}
Let’s break this down:
- CustomButton defines button properties like the name, icon, and click handler
- onClick calls our insertCustomText method
- insertCustomText gets the active editor instance and inserts HTML
Adjust the inserted content by customizing the .insertHtml() text.

Adding Multiple Buttons
To add another button, define and register another CustomButton:
var secondButton = new CustomButton({
// Button definition
});
customButton.register();
secondButton.register();
Ensure all buttons are registered by calling .register().
Result: Custom Insert Buttons
After adding JavaScript to define custom buttons, our customized rich text editor now has extra functionality:
Recap
To quickly recap the process:
- Added a Content Editor Web Part
- Referenced editor JavaScript library
- Wrote JavaScript for custom buttons
This allowed creating custom insert buttons for the rich text editor with just a few lines of code.
You can now augment any SharePoint rich text editor to better suit your needs. The possibilities are endless!
Try adding buttons to insert templates, branding, signatures, and more. Turbocharge your editors using the simple framework outlined above.
The SharePoint rich text editor allows authors to format text, add images and links, create tables, and more. This guide teaches you how to extend the editor.
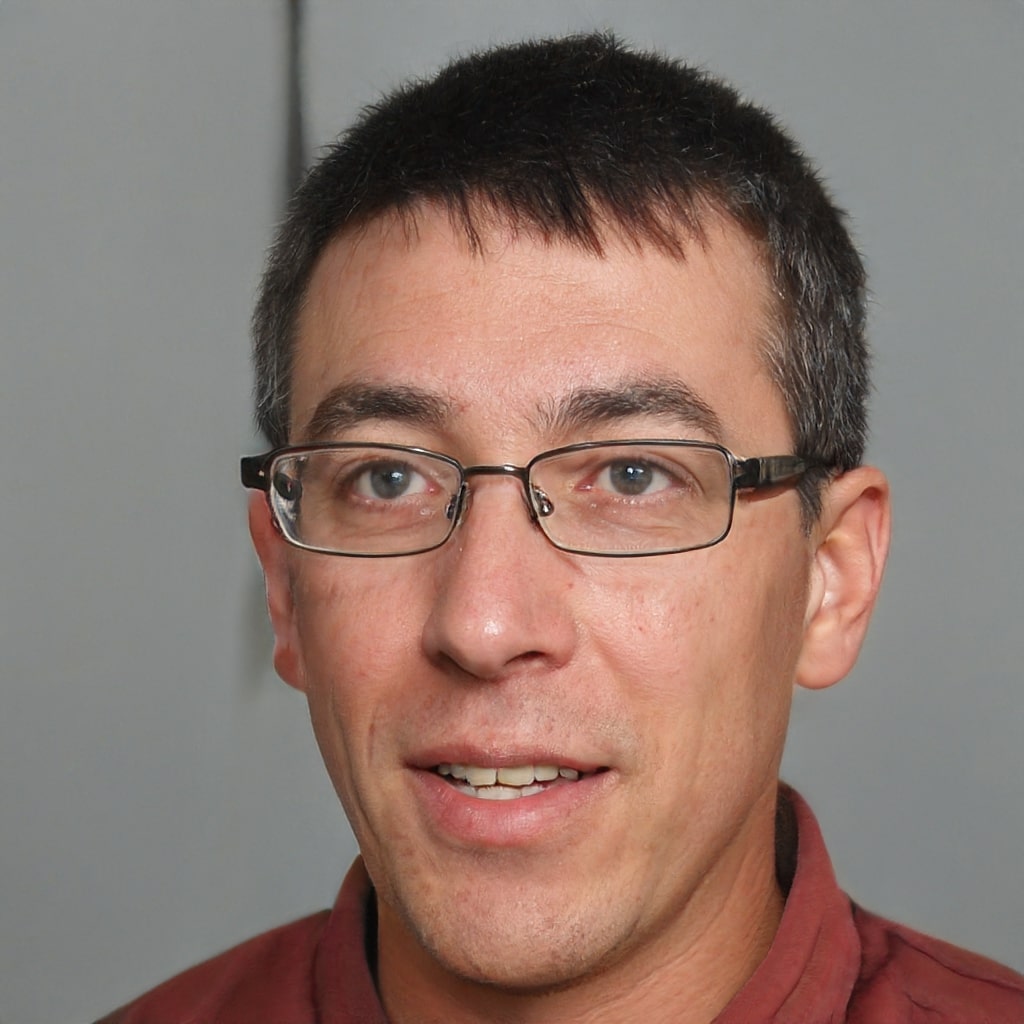